Service for generating snapshots of the composable player.
Overview
Player snapshots are an important key element of the front-end implementation. These images are often required on summary and order pages or emails, to give a quick preview of the configuration without loading the player itself.
For this purpose we have set up a snapshot service that enables the front-end to trigger a request for a snapshot to be generated and sent back. The process works as follows:
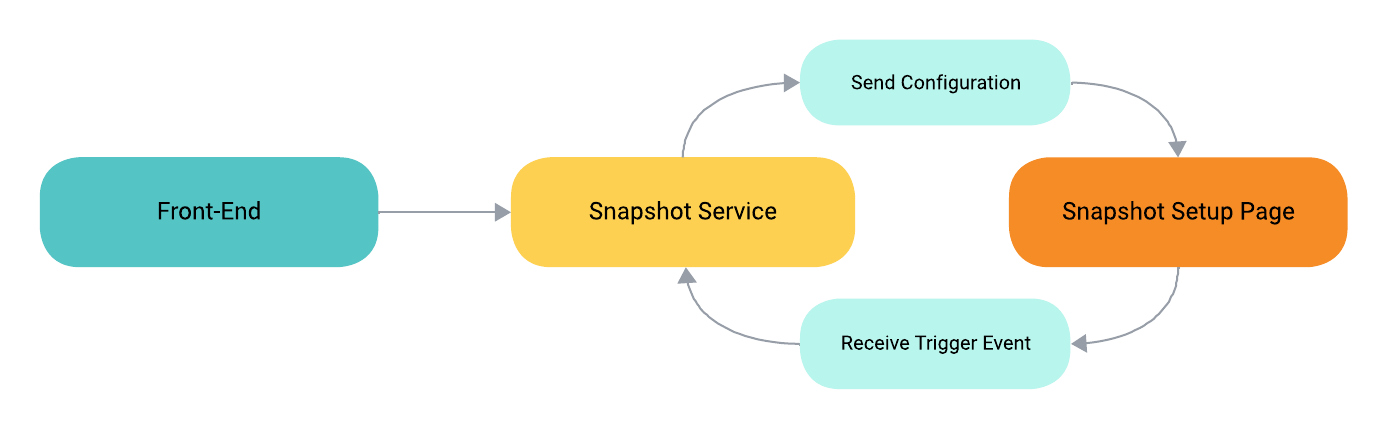
- Setup a dedicated page of the player where the player takes up the full size of the page, with the UI and icons all turned off. This page relies on query parameters to load the player for the required item with the required configuration parameters. The page will need to check when the assets have finished loading, and then trigger a special event for the service to take the snapshot.
- Send a GET request for the image to
https://snapshot.alpha.3kit.com/api/snapshot/capture
using a set of required query parameters that includes the URL of the dedicated player set up in step 1. - If the image has not yet been generated and cached, then the service will wait for the player to load and capture the image before it sends it back. This could take a little while.
Requesting a Snapshot
Snapshots can be received with a GET request from the following URL using the appropriate query parameters:
https://snapshot.alpha.3kit.com/api/snapshot/capture
https://snapshot.alpha.3kit.com/api/snapshot/capture
Query Parameters
These are required query parameters for the
Name | Type | Description | Status |
---|---|---|---|
sourceUrl | String | URL to the Snapshot Setup Page, including the configuration in query parameters | Required |
width | Number | Width of the snapshot image | Required |
height | Number | Height of the snapshot image | Required |
cacheScope | String | Cached version of the assets | Required |
Example
const sourceHost = "https://exampleconfigurator.com/snapshotPage"; //replace this with your actual source host
const sourceUrl = `${sourceHost}?Fabric=${fabricOption}&Legs=${legsOption}&ui=false`; //replace this with your actual product configuration parameters
const snapshotSearchParams = new URLSearchParams();
snapshotSearchParams.set("sourceUrl", sourceUrl);
snapshotSearchParams.set("width", `${width}`); //replace this with your actual width
snapshotSearchParams.set("height", `${height}`); //replace this with your actual height
snapshotSearchParams.set("cacheScope", "v12"); //replace this with your actual cache scope
const snapshotHost = "https://snapshot.alpha.3kit.com/api/snapshot/capture";
return (
<div>
<img
src={`${snapshotHost}?${snapshotSearchParams.toString()}`}
/>
</div>
);
Snapshot Setup Page
In order to take the snapshot, the Snapshot Service requires that you set up a dedicated web page for this purpose. These are the requirements:
- This page needs to have only the Composable Player embedded, with icons switched off, and no other UI present.
- You need to set up the page to read the incoming query parameters passed from the Snapshot Service, and apply the configuration to the player assets.
- Check that the assets have finished loading, and then trigger the following event:
document.dispatchEvent(new CustomEvent('readyForScreenshot'));